Widget API Documentation
1.Load API Script
Add following javascript file to your page.
<script src="//services.wheel-size.com/code/ws-widget.js"></script>
After that global variable WheelSizeWidgets
will be available.
2.Embed Widget
Use WheelSizeWidgets.create
method to create widget instance:
WheelSizeWidgets.create(selector, configuration)
Embeds widget's iframe into container specified by selector.
Parameters
selector (string) - css selector identifying widget container element
configuration (object) - configuration options, may contain following properties
uuid (string) required - configuration uuid
width (string|number) - widget html width
height (string|number) - widget html height
iframeAttrs (object) - standard html parameters for iframe tag
Returns
Widget instance
Notes
- If iframe element is already exist and you just want to create Widget instance,
leave absent uuid field or configuration parameter at all.
- If height field is evaluated to false, autoresize is used.
It means that iframe window will automatically adjust its height for content.
Widget instance contains following attributes:
iframe (element) - iframe element
parent (element) - iframe container element, specified by selector
config (object) - configuration object
Example usage:
<div id="ws-widget-fdbe15"></div>
<script>
var widget = WheelSizeWidgets.create('#ws-widget-fdbe15', {
uuid: 'fdbe15edbc074b59ba5efd0bd3d3ee3c',
width: 600
});
</script>
Make sure that WheelSizeWidgets.create
is called when corresponding
container element is already exists.
Another way is to create iframe element manually in html.
If you than want to use javascript API, use WheelSizeWidgets.create
method without configuration parameter.
<div id="ws-widget-fdbe15">
<iframe src="//services.wheel-size.com/widget/fdbe15edbc074b59ba5efd0bd3d3ee3c/"
width="600" height="400" frameborder="0">
</iframe>
</div>
<script>
var widget = WheelSizeWidgets.create('#ws-widget-fdbe15');
</script>
3.Widget Events
The Widget
instances have on
and off
methods for event handling.
Widget.on(type, callback)
Binds a callback function to the widget.
Parameters
type (string) required - event type
callback (function) required - event handler
Widget.off(type, callback)
Removes a previously-bound callback function from the widget.
Parameters
type (string) required - event type
callback (function) required - event handler
The API provides following events:
ready:document - fires when iframe's DOM is ready
ready:window - fires when iframe's window is loaded
activate - fires when any new tab activated
activate:tab - fires when the tab activated
tab is one of search_by_model, search_by_tire, search_by_rim
Event data contains
newTab (string) - activated tab, one of tabs
change - fires when any resource is changed
change:resource - fires when resource's select value is changed
resource is one of make, year, model, tire_width, aspect_ratio, rim_diameter, rim_width, bolt_pattern
Event data contains
resource (string) - identifier of changed resource, one of resources
value (object)
slug (string) - slug of new value, e.g. "aston-martin"
title (string) - human readable value, e.g. "Aston Martin"
Any event data also contains
tab (string) - identifier of current tab, one of tabs
Example usage:
var widget = WheelSizeWidgets.create('#ws-widget-fdbe15');
widget.on('ready:window', function(e) {
console.log('Widget is loaded');
});
widget.on('change:make', function(e) {
console.log('Make is changed. New value object is', e.data.value);
});
4.Custom Links in Search Result
Do you want to use our widget for search and insert links to your site? Look at the image below - there is plenty of places where you can embed your links.
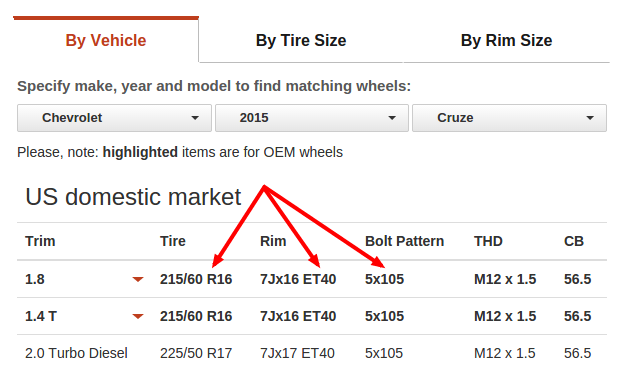
Our widget api allows to embed three type of links in each column. You can insert link before, after or instead of original content.
widget.registerUserLinks(linksObject)
linksObject is a key-options object
key (string) - place of user link, should be one of
beforeTrim, replaceTrim, afterTrim,
beforeTire, replaceTire, afterTire,
beforeRim, replaceRim, afterRim,
beforeBoltPattern, replaceBoltPattern, afterBoltPattern,
beforeThd, replaceThd, afterThd,
beforeCb, replaceCb, afterCb
options (object) - user link options, may contain following properties
href (string) required - template for link url
title (string) - template for link title
text (string) - template for link text
icon (string) - link icon
click (function) - click handler (more information see below)
clickPreventDefault (boolean) - set to true to prevent default click action
If link type is replace, text is ignored and used original value.
Otherwise one of text and icon is required.
Another way to specify click handlers is Widget.on method
User links API provides following events:
click - fires when any user link is clicked
click:column, e.g. click:trim - fires when any link in the column is clicked
click:place, e.g. click:replaceTrim - fires when link at specified place is clicked
Event data contains
column (string) - link column, e.g. "trim"
type (string) - link type, e.g. "replace"
link (object)
text (string) - interpolated link text (text template rendered with context)
href (string) - interpolated link href
context (object) - template context (list of context fields see below)
Template is a string with {{ placeholders }}.
You can use following variables in custom link templates:
trim (string), e.g. "1.8"
is_oem (boolean), e.g. true
bolt_pattern (string), e.g. "5x105"
thd (string), e.g. "M12 x 1.5"
lock_type (string), e.g. "nut"
cb (number), e.g. 56.5
rim (string), e.g. "7Jx16 ET40"
rim_diameter (number), e.g. 16
rim_width (number), e.g. 7
rim_offset (number), e.g. 40
tire (string), e.g. "215/60 R16"
tire_aspect_ratio (number), e.g. 60
tire_width (number), e.g. 215
fuel (string), e.g. "Petrol"
engine_type (string), e.g. "V6"
power.PS (number), e.g. 328
power.hp (number), e.g. 323
power.kW (number), e.g. 241
generation.name (string), e.g. "III Restyling"
generation.start_year (number), e.g. 2012
generation.end_year (number), e.g. 2015
make, year, model - objects with fields slug and title,
e.g. { slug: "chevrolet", title: "Chevrolet" }
Learn examples below for more information.
widget.registerUserFooter(linksObject)
User footer allows to insert custom content before or after button "... on Wheel-Size.com".
Possible keys: beforeButton, afterButton
Options has additional configuration field:
type (string) with two possible values: text, link (default is text)
For link type href, title, text templates make sence, for text - only text
Template context contains only make, year, model objects.
Example usage:
var widget = WheelSizeWidgets.create('#ws-widget-fdbe15');
widget.registerUserLinks({
replaceTire: {
href: 'http://www.wheel-size.com/tire/{{ tire }}/',
title: '{{ tire }} on wheel-size.com'
}
});
Each text in tire column will be replaced with the link to your site:
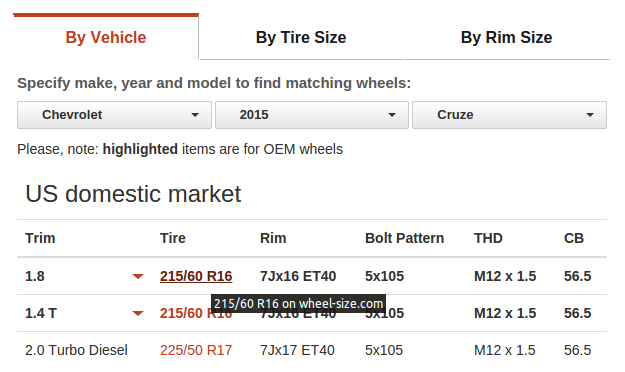
One more example:
var widget = WheelSizeWidgets.create('#ws-widget-fdbe15');
widget.registerUserLinks({
beforeTrim: {
href: 'http://www.wheel-size.com/{{ trim }}/',
icon: 'new-window',
title: '{{ trim }} on wheel-size.com',
click: function(e) {
console.log(e);
}
}
});
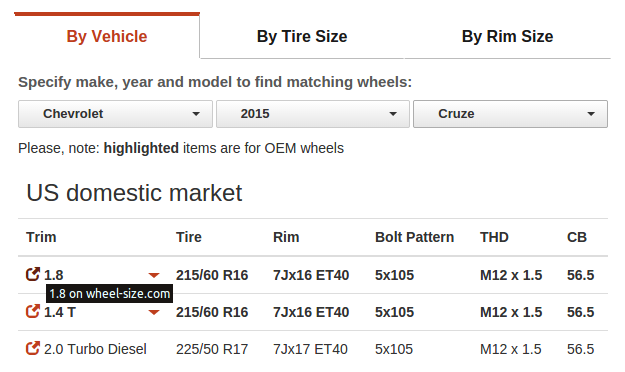
5.Live Demo
This is live demo with following code used:
var widget = WheelSizeWidgets.create('#user-links-demo', {
uuid: 'finder',
width: 800
});
widget.registerUserLinks({
beforeTrim: {
href: 'http://www.wheel-size.com/size/{{ make.slug }}/{{ model.slug }}/{{ year.slug }}/',
icon: 'new-window',
title: '{{ make.title }} {{ model.title }} {{ year.title }} on wheel-size.com'
},
replaceTire: {
href: 'http://www.wheel-size.com/tire/{{ tire }}/',
title: '{{ tire }} on wheel-size.com'
},
afterBoltPattern: {
href: 'http://www.wheel-size.com/pcd/{{ bolt_pattern }}/',
text: 'on site',
title: '{{ bolt_pattern }} on wheel-size.com'
}
});